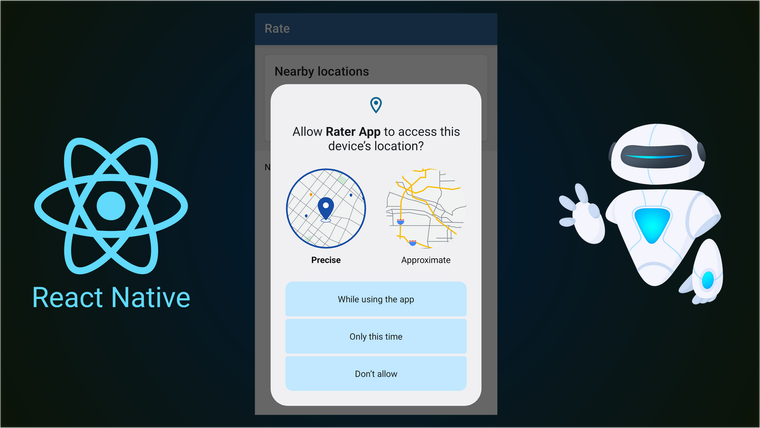
Detox and React Native: UI testing Android permission flows
I’m currently learning React Native and Expo as an alternative to native app development. To do this, I’ve been working on an app so that I can get hands-on experience with React Native app development. This includes end-to-end UI testing, using Detox.
I was surprised that Detox doesn’t have an API to interact with permissions. The
device.launchApp
function does have a permissions
field, but this only works
on iOS and runs on app start-up - it doesn’t allow you to test the actual user
flow.
In this article, I will explain how you can do end-to-end testing with Android permissions, including simulating user interaction with the Android permission request modal.
Read more of "Detox and React Native: UI testing Android permission flows"