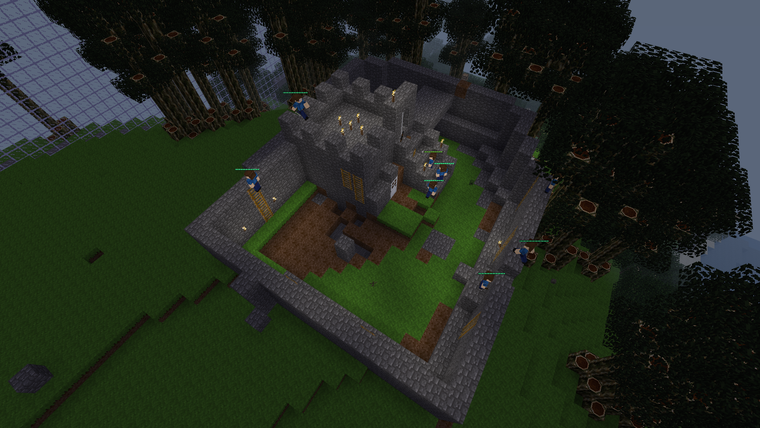
Ten years of Capture The Flag
Ten years ago today, I uploaded the first version of Capture The Flag. Capture The Flag is a multiplayer game where two teams of players battle to claim the other team’s flag whilst defending their own. Capture The Flag is played in a destructible voxel environment, allowing players to build defences and place traps.
Capture The Flag started life as a persistent kingdoms game but quickly pivoted to a match-based team game. It was developed iteratively, taking into account player feedback. I hosted a server for the game for many years and a community formed around it. In 2021, I handed over the reins to CTF to very capable hands; it remains Minetest’s most popular server to this day.
This article covers the history of CTF, the lessons I learned, and the changes I made along the way.