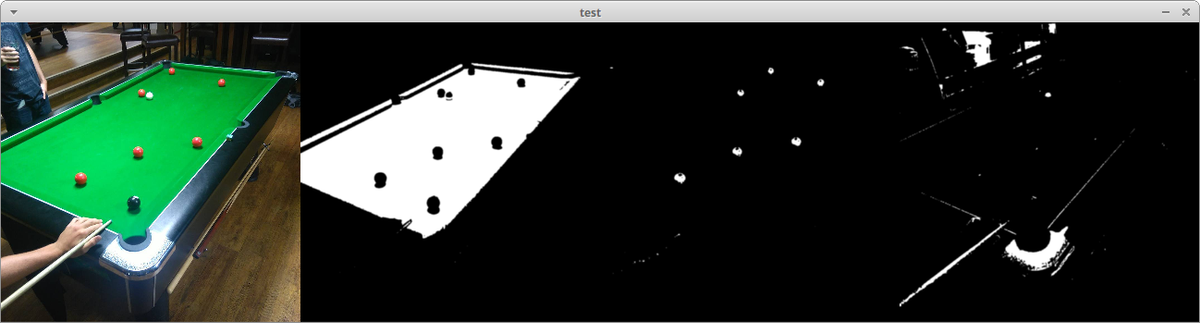
I created a very short C++ snippet to accumulate a series of `Mat`s into
a single `Mat` strip. It works like `acc = acc + m` - a new mat is added to the
accumulator each time, then stored in the accumulator again.
I created a very short C++ snippet to accumulate a series of Mat
s into
a single Mat
strip. It works like acc = acc + m
- a new mat is added to the
accumulator each time, then stored in the accumulator again.
#pragma once
#include <opencv2/opencv.hpp>
#include <opencv2/core/core.hpp>
#include <opencv2/imgcodecs.hpp>
#include <assert.h>
using namespace cv;
class MatStrip
{
public:
Mat current;
float scale;
MatStrip(Mat start, float scale=0.3f):
scale(scale)
{
resize(start, current, Size(start.cols * scale, start.rows * scale), 1.0, 1.0, INTER_CUBIC);
}
void add(const Mat &in_o)
{
Mat in;
resize(in_o, in, Size(in_o.cols * scale, in_o.rows * scale), 1.0, 1.0, INTER_CUBIC);
assert(in.type() == current.type());
Size sz1 = current.size();
Size sz2 = in.size();
Mat im3(sz1.height, sz1.width+sz2.width, current.type());
Mat left(im3, Rect(0, 0, sz1.width, sz1.height));
current.copyTo(left);
Mat right(im3, Rect(sz1.width, 0, sz2.width, sz2.height));
in.copyTo(right);
current = im3;
}
};
Comments