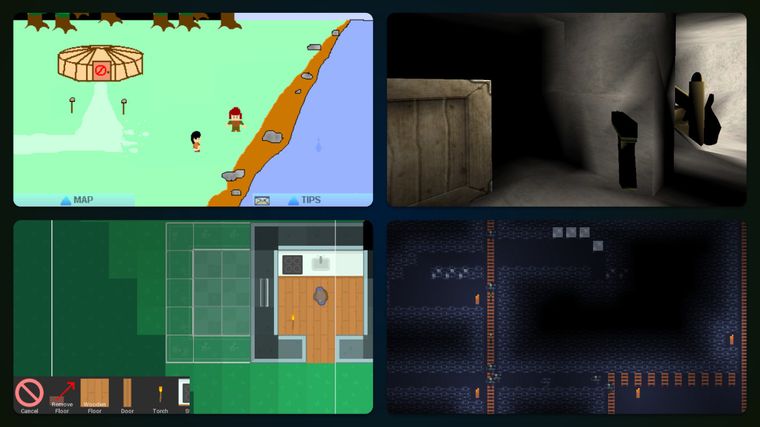
Make games not engines: why I'll be using Godot engine
One of the reasons I learned how to program was to make games. Games are a unique form of creative medium, combining art, interactive storytelling, and vibrant worlds. But as a software engineer, it’s easy to lose sight of my goals and get trapped by the technical details. It’s common for software engineers in game dev to roll their own engine, which I believe reduces productivity and is ultimately a distraction to making a game.
Note that I’m not just referring to making reusable or generic game engines; for this article, I consider using low-level technology like OpenGL, SFML, or SDL to make games to include the act of rolling your own game engine, even if the focus is specific. It’s more manageable, but you still end up reinventing the wheel and having to solve many of the same problems.
There are plenty of other articles about whether or not to make your own game engine. This article is personal to me; it’s an exploration of my journey in game dev, a discussion of what motivates me, and a promise for the future.
Read more of "Make games not engines: why I'll be using Godot engine"